Human beings do not live in the objective world alone, nor alone in the world of social activity as ordinarily understood, but are very much at the mercy of the particular language which has become the medium of expression for their society. It is quite an illusion to imagine that one adjusts to reality essentially without the use of language and that language is merely an incidental means of solving specific problems of communication or reaction. The fact of the matter is that the real world' is to a large extent unconsciously built up on the language habits of the group... We see and hear and otherwise experience very largely as we do because the language habits of our community predispose certain choices of interpretation."
Edward Sapir (quoted in [Whorf 1956])This quote emphasizes the fact that the languages we speak directly influence the way in which we view the world. This is true not only for natural languages, such as the kind studied by the early twentieth century American linguists Ed- ward Sapir and Benjamin Lee Whorf, but also for artificial languages such as those we use in programming computers.
Excerpt from Oregon State book, OOP Thinking Chapter 1)
How do we get our students to start thinking about Object Oriented Programming while still teaching procedural coding practice?
Object Oriented thinking, a term that we have adopted in our classroom and curriculum, is a term that means moving from procedural thinking in coding basics into a more object/method process of thinking. Object Oriented programming concepts such as classes and instances are challenging topics for beginners to comprehend, but by reframing and helping students identify patterns, you can make learning OOP easier. Object Oriented thinking is a great way to get students to start thinking about Python objects, methods, and jobs in preparation for the transition into more complex OOP code.
Understanding Object Oriented Programming as a new coder has always been something that has failed to make sense to me. I can copy it. I can read about it. However, when it comes to writing classes or preparing my students for more OOP, I struggle to do it. Finding ways to teach students why a sprite is a way it is with a dunder init function, or how to think about writing classes without just copying someone's code is difficult. Moreover, I do not think I am alone.
When I first started coding, I googled OOP. I read a lot. I know Python is an OOP language, a language based on objects. Got it! When writing code from books, readers are instantly thrust into copying classes of rockets or other objects to move around a screen. You can replace class names and then call the code your own, but how much is really learned?
How much of what is copied makes sense enough to write an original program? I would wager that most students do not get it.
"The mind unlearns with difficulty what it has long learned." -Seneca
Objects have methods, and different instances of objects exist.
Creating a new class creates a new type of object. This allows for new instances of that type to be made.
The DRY method, don’t repeat yourself, of using Classes and instances allows us to limit repetition within code, and reduce redundancy.
We learn something. We use it daily. It becomes second nature and it makes sense to us. Therefore, it must make sense to everyone, right?
Wrong!
OOP languages make sense to many developers or lifelong coders but it is often difficult to find ways to explain it to new coders because it is difficult to "unsee it once you learn something. " And sentences like this one from, Geeks for Geeks make no sense to a beginner but seem very logical to an experienced coder.
"Creating a new class creates a new type of object, allowing new instances of that type to be made. Each class instance can have attributes attached to it for maintaining its state. Class instances can also have methods (defined by its class) for modifying its state."
It is difficult to see what new learners are unable to see. It is often hard to remember and teach the "stuck" feeling when you cannot remember what kept you from not 'seeing' the objects in the first place. A long-term coder who has mastered OOP concepts sees the patterns while a new coder fails to make the connection.
It wasn't until I started to see the patterns and connections that classes and methods made sense.
How do we get our students to start thinking about Object-Oriented Programming while helping them to transfer to a less procedural coding practice?
Teaching students about classes is not as easy as we think it to be. It is not a simple ' I code, you code'; they got it. It is a lot different than the procedural paradigm of coding. After 4-6 weeks of coding, students can generally write basic original programs on their own. The procedural method is easy for students to understand and mimic because it has been learned before, in science, in narrative writing, and in other courses. Write what you need to do from top to bottom and read it from left to right.
You see it in a beginner's code every day and as they progress to using more complex code in their turtle programs.
What if, during the early stages of teaching students to code, procedural instruction of coding is intertwined with OOP thinking?
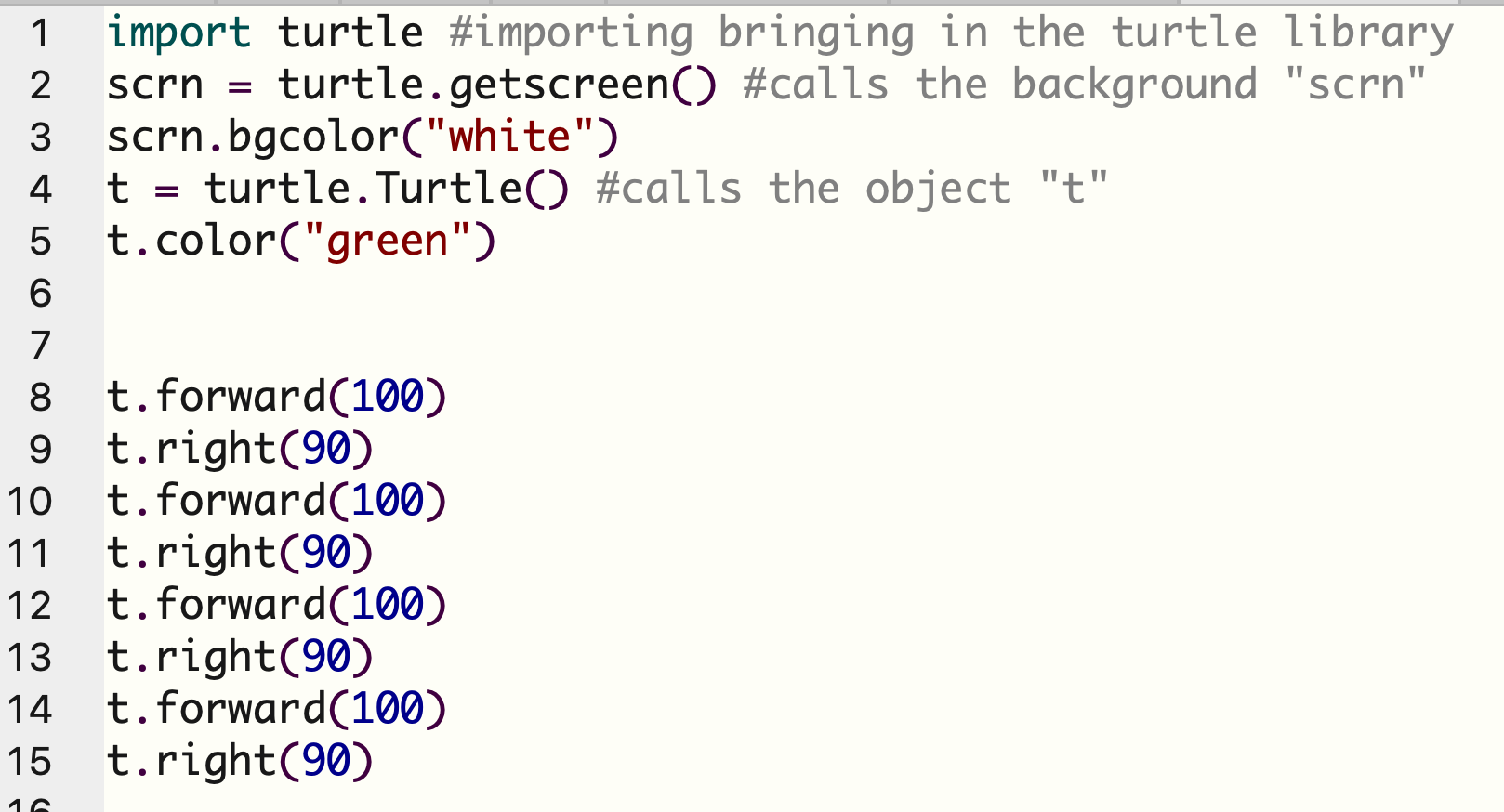
To make real use of object oriented programming, we often need to teach students how to see the world in a new way. The way a programmer thinks about solving a problem is typically the way that the code will be developed. Transitioning the way we teach problem solving and designing code can help a student feel more comfortable with object oriented programming.
By introducing this way of thinking, students can begin to imagine pieces of code as objects that have jobs(procedures) and methods of doing their job. Furthermore, more complex concepts, such as objects, are introduced, and refactoring code throughout instruction can help students use terminology and skills necessary for OOP. Starting students with the refactoring of procedural code and identifying more object-oriented thinking can help students "unlearn" the need to make all code procedural and understand that they may need to use OOP to do cool things.
This video from Linkedin, offers insight into how students can see code in a more procedural cookbook way versus through objects and methods. These metaphors help to create mental images for the two thought processes of coders. Its simplicity and visuals offer learners and teachers a good starting point for Object Oriented thinking. Moving between procedural and object oriented paradigms happens as the code becomes complex and the need to reduce redundancy becomes obvious.
Four tenets to designing lessons that promote object oriented thinking
Teachers put a lot of time into designing lessons that help to develop skills in students. The collective skills that a coder needs to develop in order to produce original code are complex. It is not a simple task and requires a wide variety of skills that are intertwined. A few basic tenets can be used to help students develop a more object-oriented way of thinking.
The first tenet is to teach students how to read code both "top to bottom-left to right" as well as "right to left and bottom to top."
For example:
greeting = "hello"
Procedural:
The variable "greeting" is assigned to the string "hello".
OOP thinking:
Thinking: The string "hello" is assigned to the object called "greeting"
title_greeting = greeting.title()
For example:
Procedural:
Reassign the greeting variable to another variable name and make it title case.
OOP thinking:
Apply the title method to the String object to capitalize greeting and assign it to "title_greeting"
The second tenet is to use language that will help students communiate and develop a more object oriented way of thinking.
The information in provided in inline quotes and is repeated to the students. It is used to reinforce the language of object oriented coding.
For example:
Procedural:
import turtle as t #always write this to import turtle
t.forward(100) #make t move
OOP thinking:
import turtle #import turtle library with all the functions and methods
bob = turtle.Turtle() #assign the turtle object turtle from the Turtle class to an object named "bob"
bob.forward(100) #apply the method .forward() to make turtle move give it parameter for distance
I like to tell my students:
"The turtle module is a library full of all kinds of functions and methods that some cool coder programmed for us. These cool coders wrote all kinds of libraries with objects, classes, and methods, so that all we need to know how to do is read the documentation and write the essential lines of code.
I follow up with:
- Different ways of doing something to an object exist in code.
- We can apply functions and methods to make an object react.
- Objects exist in Python, and we apply methods to them.
- .lower(), .append(), .keys()
The third tenet is to point out the patterns and repeat often.
Throughout the course, I like to help students see patterns.
The patterns that exist in code are beautiful. Lining up different types of objects and methods in code can help students identify patterns easier. Using color and writing on whiteboards also helps. The basics of code and identifying a few easy patterns can help students understand more code complexities later on.
For example:
Methods are applied to the objects. Students can see this with string, list and dictionary methods easily.
The fourth tenet is to use mental images and comparisons with ordinary objects.
- Objects can be different things, just like objects in our lives.
- strings, lists, dictionaries, turtles are the same as chair, car, cookies, and games
- There can be many objects of the same data type.
- We can put many lists, strings, and turtles in our code. Just like we can have many types of chairs, cars, cookies, and games.
Helping students to start visualizing, and comprehending the patterns in code is a great way to help them comprehend OOP.
These four tenets are a good start for developing OOP thinking. There are many more techniques such as desiging and developing solutions that can also help with the mindset.
In addition, keeping these questions in mind as you develop lessons can help you plan more effective lessons for more object oriented thinkers:
- How can I explain things better to my students?
- How do I ensure that eventually, they will "see" objects and never unsee them?
- When do my students need to know and understand classes and instances?
- When is the right time to teach them this?
What other questions exist? How do you you get your students to start thinking about Object Oriented Programming while still teaching procedural coding practice?
“Instance Method in Python.” GeeksforGeeks, 2 July 2020, www.geeksforgeeks.org/instance-method-in-python/.
OOP Intro Chapter 1 Thinking Object Oriented. http://web.engr.oregonstate.edu/~budd/Books/oopintro3e/info/chap01.pdf.
Stone, Olivia Chiu. “Object Oriented Thinking - Python Video Tutorial: LinkedIn Learning, Formerly Lynda.com.” LinkedIn, 27 Nov. 2018, www.linkedin.com/learning/programming-foundations-object-oriented-design-3/object-oriented-thinking.